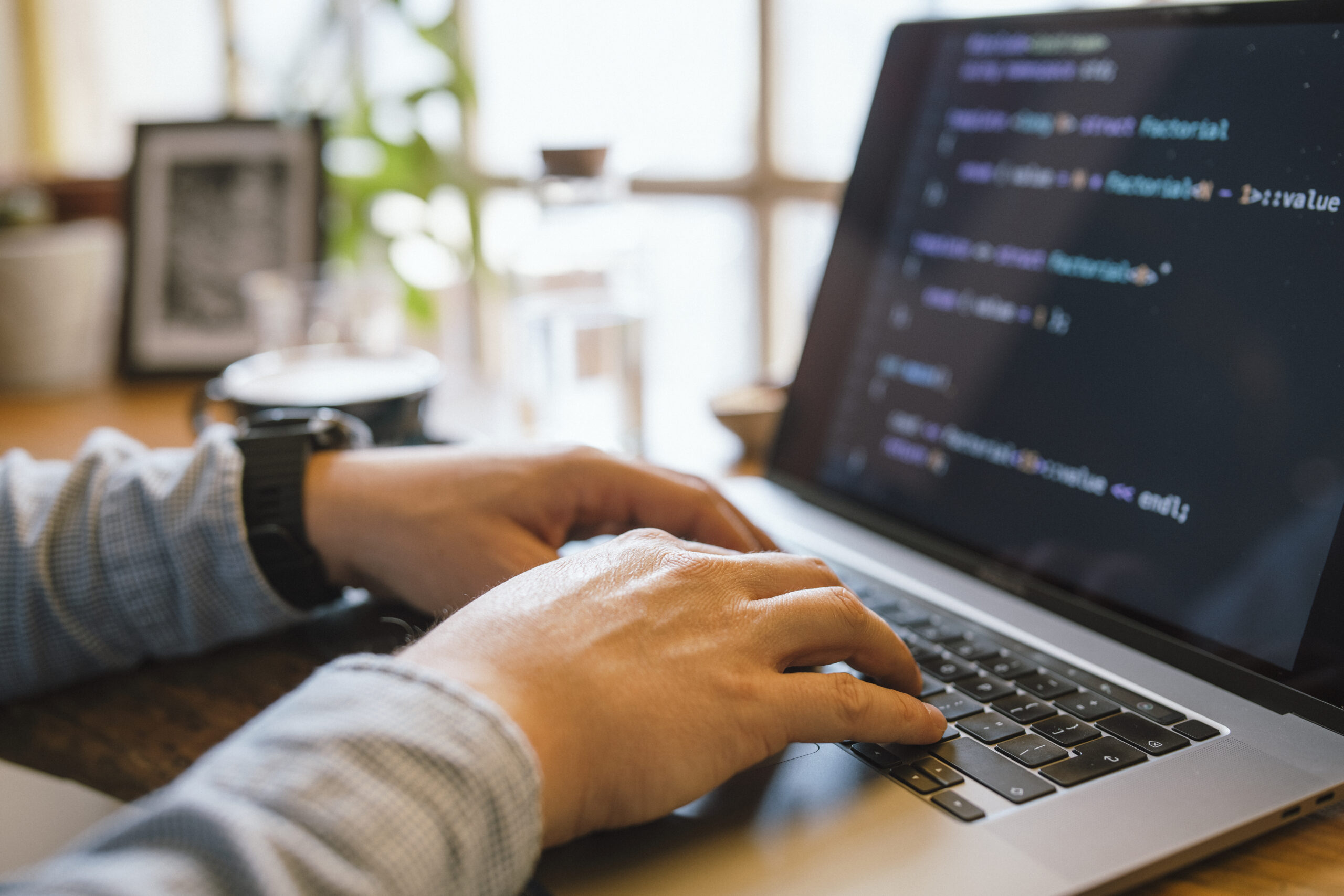
Debugging is Among the most important — nevertheless typically missed — abilities inside a developer’s toolkit. It is not almost repairing damaged code; it’s about comprehending how and why items go Improper, and Finding out to Assume methodically to unravel problems efficiently. Regardless of whether you're a rookie or maybe a seasoned developer, sharpening your debugging competencies can preserve hrs of stress and substantially transform your productiveness. Here's several strategies to aid builders degree up their debugging recreation by me, Gustavo Woltmann.
Grasp Your Instruments
Among the list of fastest techniques developers can elevate their debugging skills is by mastering the applications they use everyday. While crafting code is one part of enhancement, knowing ways to interact with it effectively during execution is Similarly significant. Modern-day advancement environments appear equipped with powerful debugging abilities — but several developers only scratch the area of what these applications can do.
Take, for instance, an Built-in Growth Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications help you set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code on the fly. When applied accurately, they let you notice specifically how your code behaves all through execution, which is priceless for monitoring down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for front-end builders. They enable you to inspect the DOM, monitor community requests, see authentic-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, resources, and network tabs can switch frustrating UI challenges into manageable jobs.
For backend or method-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep Regulate over managing processes and memory administration. Studying these equipment can have a steeper Finding out curve but pays off when debugging performance troubles, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfortable with Edition Regulate systems like Git to comprehend code background, uncover the precise instant bugs were being released, and isolate problematic modifications.
In the end, mastering your applications means going past default options and shortcuts — it’s about establishing an personal expertise in your growth natural environment to make sure that when challenges crop up, you’re not shed at the hours of darkness. The better you understand your equipment, the more time you may spend solving the actual challenge in lieu of fumbling by the method.
Reproduce the challenge
The most essential — and sometimes forgotten — techniques in productive debugging is reproducing the challenge. Ahead of jumping in to the code or creating guesses, builders want to create a constant environment or state of affairs wherever the bug reliably appears. Devoid of reproducibility, repairing a bug will become a video game of probability, typically leading to squandered time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire thoughts like: What actions brought about the issue? Which ecosystem was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more detail you have got, the less complicated it gets to be to isolate the precise problems under which the bug happens.
As soon as you’ve collected plenty of info, seek to recreate the trouble in your neighborhood surroundings. This could signify inputting the identical details, simulating comparable person interactions, or mimicking method states. If The problem seems intermittently, look at writing automated checks that replicate the edge situations or point out transitions involved. These checks not just enable expose the problem but in addition reduce regressions in the future.
Often, The difficulty could possibly be ecosystem-particular — it would materialize only on specified functioning systems, browsers, or underneath particular configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating these types of bugs.
Reproducing the problem isn’t only a phase — it’s a mentality. It requires persistence, observation, plus a methodical tactic. But after you can persistently recreate the bug, you happen to be now midway to correcting it. Which has a reproducible scenario, You may use your debugging applications more effectively, check possible fixes securely, and talk a lot more Plainly with the staff or people. It turns an summary grievance into a concrete problem — and that’s wherever builders thrive.
Go through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when one thing goes Improper. Instead of seeing them as frustrating interruptions, builders really should understand to deal with error messages as direct communications from the procedure. They normally show you just what exactly occurred, wherever it occurred, and occasionally even why it happened — if you understand how to interpret them.
Commence by studying the information thoroughly As well as in total. Numerous builders, particularly when under time force, glance at the main line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like yahoo — read and recognize them initial.
Crack the error down into areas. Is it a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These inquiries can guide your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to recognize these can greatly quicken your debugging approach.
Some faults are obscure or generic, As well as in those situations, it’s very important to examine the context through which the mistake occurred. Test related log entries, input values, and up to date variations in the codebase.
Don’t forget about compiler or linter warnings both. These generally precede larger difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive resources within a developer’s debugging toolkit. When employed properly, it offers true-time insights into how an application behaves, supporting you recognize what’s occurring underneath the hood without having to pause execution or action from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what degree. Popular logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts all through development, Facts for typical gatherings (like profitable commence-ups), WARN for opportunity difficulties that don’t split the appliance, ERROR for real issues, and FATAL when the procedure can’t go on.
Prevent flooding your logs with abnormal or irrelevant details. Far too much logging can obscure vital messages and slow down your program. Concentrate on key events, point out alterations, enter/output values, and demanding decision factors within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and performance names, so it’s easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you monitor how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in manufacturing environments where by stepping by means of code isn’t probable.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging technique, you'll be able to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and reliability within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To successfully discover and fix bugs, builders need to solution the method just like a detective resolving a mystery. This state of mind can help stop working advanced issues into manageable elements and comply with clues logically to uncover the foundation cause.
Begin by collecting proof. Look at the signs or symptoms of the problem: error messages, incorrect output, or functionality challenges. Just like a detective surveys a crime scene, gather as much pertinent data as you are able to with out jumping to conclusions. Use logs, check circumstances, and user reviews to piece with each other a clear image of what’s happening.
Following, kind hypotheses. Question by yourself: What may be triggering this conduct? Have any alterations not long ago been designed to your codebase? Has this issue happened in advance of beneath related situations? The objective is to slender down opportunities and recognize possible culprits.
Then, examination your theories systematically. Attempt to recreate the condition in a very controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out close notice to small information. Bugs frequently disguise inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular mistake, or simply a race issue. Be complete and individual, resisting the urge to patch The difficulty without having fully comprehension it. Temporary fixes may possibly hide the true issue, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and support others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden difficulties in complex techniques.
Produce Checks
Writing tests is one of the best solutions to help your debugging abilities and All round enhancement efficiency. Tests not just aid catch bugs early but in addition function a security Internet that provides you assurance when earning adjustments to the codebase. A very well-analyzed software is simpler to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with unit checks, which focus on individual capabilities or modules. These compact, isolated checks can immediately expose no matter if a certain bit of logic is Functioning as envisioned. Every time a take a look at fails, you quickly know the place to search, substantially decreasing the time spent debugging. Device assessments are Specifically handy for catching regression bugs—troubles that reappear right after previously being fastened.
Following, integrate integration checks and conclusion-to-conclude exams into your workflow. These help make sure many portions of your application do the job jointly easily. They’re particularly practical for catching bugs that arise in complicated units with a number of components or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Consider critically about your code. To test a feature thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This standard of understanding Obviously leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing take a look at that reproduces the bug may be a strong starting point. After the take a look at fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is fixed. This strategy makes sure that a similar bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a annoying guessing activity into a structured and predictable procedure—supporting you capture much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your brain, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be less productive at issue-solving. A brief stroll, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your focus. Many builders report obtaining the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance avert burnout, Specifically throughout longer debugging classes. Sitting before a display screen, mentally caught, is not only unproductive but will also draining. Stepping away enables you to return with renewed Electrical power plus a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a very good general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to a lot quicker and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak spot—it’s a sensible method. It offers your Mind Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply a temporary setback—It really is a possibility to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can educate you a thing important if you take some time to mirror and assess what went Erroneous.
Commence by asking by yourself a number of critical thoughts as soon as the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code evaluations, or logging? The answers usually reveal blind spots with your workflow or comprehension and make it easier to Make more robust coding behaviors transferring forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll begin to see designs—recurring problems or common mistakes—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short create-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. After all, many of the very best builders aren't those who create great code, but those that repeatedly discover from their faults.
In the end, Just about every bug you repair adds a completely new layer in your website talent established. So up coming time you squash a bug, have a moment to mirror—you’ll occur away a smarter, far more able developer as a result of it.
Summary
Improving upon your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be much better at Whatever you do.